thresholdcv
Applies a threshold to each element of the given image, handle, resulting in a grayscale image, R, whose pixels represent the elements exceeding the threshold.
Syntax
[computedthreshold, R] = thresholdcv(handle, threshold, max, type)
Inputs
- handle
- Handle of an image.
- threshold
- Real vector of [width height] representing the dimensions of R.
- fx
- Threshold value.
- max
- Maximum value to use with type value of 8 or 16.
- type
- Threshold type. Valid values are:
- 0
- R(x,y) = max if handle(x,y) > threshold and 0 otherwise.
- 1
- R(x,y) = 0 if handle(x,y) > threshold and max otherwise.
- 2
- R(x,y) = threshold if handle(x,y) > threshold and handle(x,y) otherwise.
- 3
- R(x,y) = handle(x,y) if handle(x,y) > threshold and 0 otherwise.
- 4
- R(x,y) = 0 if handle(x,y) > threshold and handle(x,y) otherwise.
- 8
- Uses Otsu algorithm for optimal threshold.
- 16
- Uses Triangle algorithm for optimal threshold.
Outputs
- computedthreshold
- Computed threshold value.
- R
- Handle of the resized image.
Example
handle = imreadcv('bird4.jpg', 0);
[computedthreshold, mask] = thresholdcv(handle, 10, 255, 0);
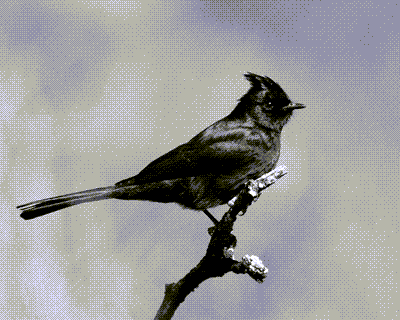
Figure 1. Input image
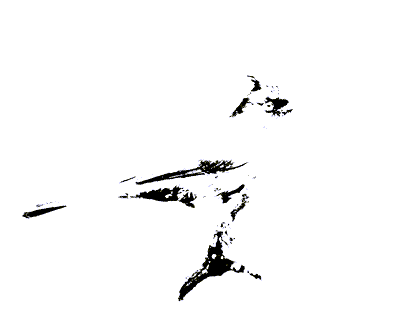
Figure 2. Mask
src = imreadcv('thresholdcv_fig1.png');
figure(1);
imshowcv(src);
thresh = 0;
maxValue = 255;
[computedthreshold, mask] = thresholdcv(src, thresh, maxValue, 0); %Binary threshold
figure(2);
imshowcv(mask);
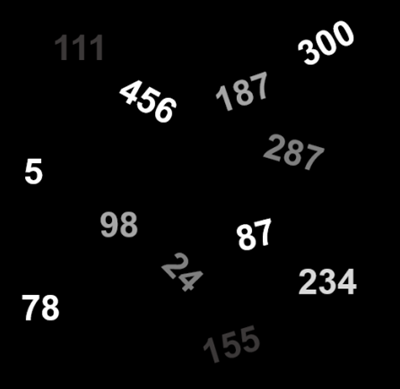
Figure 3. Input image
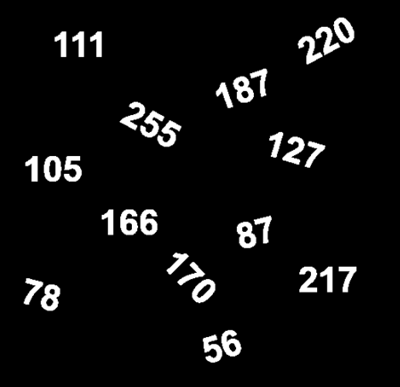
Figure 4. Mask
src = imreadcv('thresholdcv_fig1.png');
figure(1);
imshowcv(src);
thresh = 127;
maxValue = 255;
[computedthreshold, mask] = thresholdcv(src, thresh, maxValue, 0); %Binary threshold
figure(2);
imshowcv(mask);
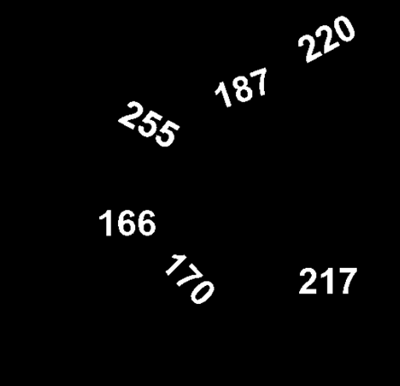
Figure 5. Mask
src = imreadcv('thresholdcv_fig1.png');
figure(1);
imshowcv(src);
thresh = 127;
maxValue = 150;
[computedthreshold, mask] = thresholdcv(src, thresh, maxValue, 0); %Binary threshold
figure(2);
imshowcv(mask);
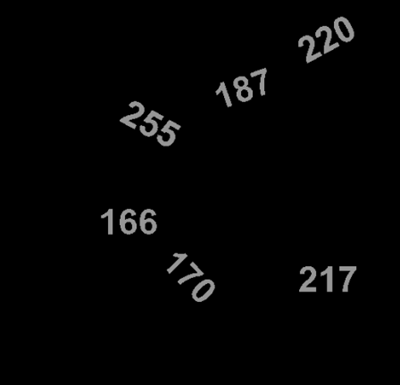
Figure 6. Mask
src = imreadcv('thresholdcv_fig1.png');
figure(1);
imshowcv(src);
thresh = 0;
maxValue = 255;
[computedthreshold, mask] = thresholdcv(src, thresh, maxValue, 1); %Inverse binary threshold
figure(2);
imshowcv(mask);
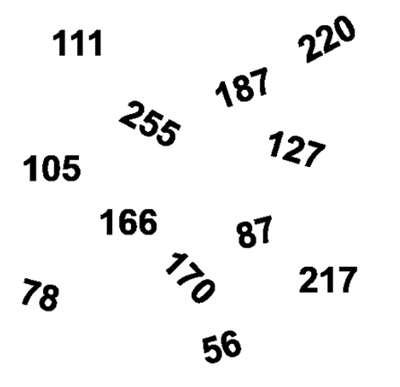
Figure 7. Mask
src = imreadcv('thresholdcv_fig1.png');
figure(1);
imshowcv(src);
thresh = 150;
maxValue = 255;
[computedthreshold, mask] = thresholdcv(src, thresh, maxValue, 2); %Inverse binary threshold
figure(2);
imshowcv(mask);
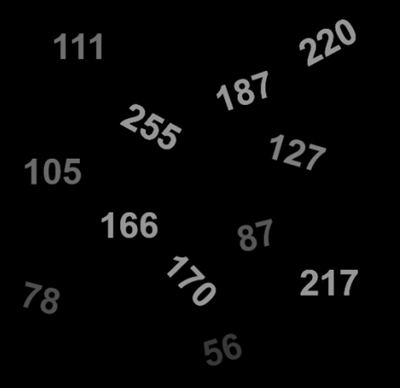
Figure 8. Mask