Entities and Solver Interfaces
A solver interface is made up of a template and a FE-input reader.
Altair HyperMesh Entities
- Data names
- Part of the entity data structure itself and are available to all instantiations of the entity regardless if the entity has an associated card image or not.
- Attributes
- Additional data, defined in a solver interface template, which are necessary to store solver specific data for a card image associated with an entity.
Solver Interfaces
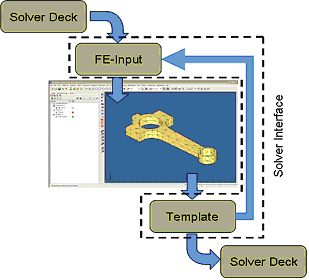
Templates
(1) | (2) | (3) | (4) | (5) | (6) | (7) | (8) | (9) | (10) |
---|---|---|---|---|---|---|---|---|---|
MAT1 | MID | E | G | NU | RHO | A | TREF | GE | |
ST | SC | SS |
The Engineering Solutions material named entity has data names of name and ID. Therefore the template would also have to define attributes for E, G, NU, RHO, A, TREF, GE, ST, SC, and SS in order to completely define and store the OptiStruct MAT1 solver card within a Engineering Solutions material named entity with a MAT1 card image. Templates define attributes using the *defineattribute() command. The example template code uses the *defineattribute() command to define these attributes.
In order to associate the OptiStruct MAT1 solver card to the Engineering Solutions material named entity with a MAT1 card image the template would contain a *materials(MAT1) definition block to define this association. Within the *materials(MAT1) definition block a MAT1 card image would be defined using a *beginmenu() definition block . This *beginmenu() definition block is read every time a materials named entity with a MAT1 card image is card edited using the card editor within Engineering Solutions. In addition, an export format for the OptiStruct MAT1 solver card would be defined using a *format() definition block within the *materials(MAT1) definition block. This *format() definition block is read every time an export of the Engineering Solutions database is requested which contains a materials named entity with a MAT1 card image. Example template code which performs these definitions for the OptiStruct MAT1 solver card is given.
In the example below you can find this example template code in [Install Directory]\hm\examples\templates\ExampleTemplate.tpl.
*codename(ExampleTemplate,100)
//MAT1 Attributes
*defineattribute(MAT1,1,integer,none)
*defineattribute(E,2,real,none)
*defineattribute(G,3,real,none)
*defineattribute(NU,4,real,none)
*defineattribute(RHO,5,real,none)
*defineattribute(A,6,real,none)
*defineattribute(TREF,7,real,none)
*defineattribute(GE,8,real,none)
*defineattribute(ST,9,real,none)
*defineattribute(SC,10,real,none)
*defineattribute(SS,11,real,none)
//Materials Named Entity - MAT1 Card Image and Export Format
*materials(MAT1)
//MAT1 Card Image
*beginmenu()
*menustring("MAT1 ")
*menufield("ID",integer,id,8)
*menufield("E",real,$E,8)
*menufield("G",real,$G,8)
*menufield("NU",real,$NU,8)
*menufield("RHO",real,$RHO,8)
*menufield("A",real,$A,8)
*menufield("TREF",real,$TREF,8)
*menufield("GE",real,$GE,8)
*menulineend()
*menustring(" ")
*menufield("ST",real,$ST,8)
*menufield("SC",real,$SC,8)
*menufield("SS",real,$SS,8)
*menulineend()
*endmenu()
//MAT1 Export Format
*format()
*string("MAT1 ")
*field(integer,id,8)
*field(real,$E,8)
*field(real,$G,8)
*field(real,$NU,8)
*field(real,$RHO,8)
*field(real,$A,8)
*field(real,$TREF,8)
*field(real,$GE,8)
*end()
*string(" ")
*field(real,$ST,8)
*field(real,$SC,8)
*field(real,$SS,8)
*end()
*output()
FE-Input Readers
FE-input readers perform the function of reading solver decks and importing solver cards into the appropriate entities with the appropriate card images, data names, and attributes set as defined by the template. Furthermore, FE-input readers require template attribute definitions to perform their tasks.
In the FE-Input code example below, you can find this example template code in [Install Directory]\hm\examples\feinput\ExampleFEInput.cxx.
#include <iostream>
#include <fstream>
#include <cstring>
#include "hmlib.h"
#include "hminlib.h"
using namespace std;
//Material Data Structure
int nummaterials;
struct materials {
char name[12];
int id;
double E;
double G;
double NU;
double RHO;
double A;
double TREF;
double GE;
double ST;
double SC;
double SS;
} material[100];
//Function Prototypes
int get_data(char *fileptr);
entityfunctionptr HM_getfunction(int function, HM_entitytype entities);
int HM_getMaterials();
int main(int argc, char *argv[])
{
/* The main function calls get_data to process the data in the solver deck,
initializes HyperMesh, sets the solver to 100 (the same number defined in
the template), reads the model and passes material data structures to HyperMesh,
and finally closes the connection between HM and the FE-input reader. */
get_data(argv[1]);
HMIN_init("ExampleFEInput", "10.0", argc, argv);
HMIN_setsolver(100);
HMIN_readmodel(HM_getfunction);
HMIN_close();
return(0);
}
int get_data(char *fileptr)
{
/* This function opens a solver deck defined as the first argument on the
input line and reads the solver deck for MAT1 cards. If a MAT1 card is found
then the MAT1 solver card is read and a material data structure is populated. */
ifstream infile;
char token[9];
char line[128];
//Open Solver Deck
infile.open(fileptr, ios::in);
if (infile.fail())
return(1);
//Read Solver Deck for MAT1 Solver Cards and Populate Material Data Structure
nummaterials = 0;
while (!infile.eof())
{
infile.get(token, 9);
if (strcmp(token, "MAT1 ") == 0)
{
//Name
strcpy_s(material[nummaterials].name, "material");
//id
infile.get(token, 9);
material[nummaterials].id = atoi(token);
//E
infile.get(token, 9);
material[nummaterials].E = atof(token);
//G
infile.get(token, 9);
material[nummaterials].G = atof(token);
//NU
infile.get(token, 9);
material[nummaterials].NU = atof(token);
//RHO
infile.get(token, 9);
material[nummaterials].RHO = atof(token);
//A
infile.get(token, 9);
material[nummaterials].A = atof(token);
//TREF
infile.get(token, 9);
material[nummaterials].TREF = atof(token);
//GE
infile.get(token, 9);
material[nummaterials].GE = atof(token);
infile.get();
//Blank Field
infile.get(token, 9);
//ST
infile.get(token, 9);
material[nummaterials].ST = atof(token);
//SC
infile.get(token, 9);
material[nummaterials].SC = atof(token);
//SS
infile.get(token, 9);
material[nummaterials].SS = atof(token);
infile.get();
nummaterials++;
}
else
infile.getline(line, sizeof(line));
}
return(0);
}
entityfunctionptr HM_getfunction(int function, HM_entitytype entities)
{
/* This user-defined function is passed into hminlib and is
used by hminlib to find all of the user-defined functions
which perform reading and information passing. Note
that if a user-defined function is not required, this function
must return NULL. */
switch (function)
{
case HMIN_OPENFUNCTION:
break;
case HMIN_ENTITYOPENFUNCTION:
break;
case HMIN_ENTITYGETFUNCTION:
switch (entities)
{
case HM_ENTITYTYPE_NULL:
break;
case HM_ENTITYTYPE_CARDS:
break;
case HM_ENTITYTYPE_SYSTCOLS:
break;
case HM_ENTITYTYPE_SYSTS:
break;
case HM_ENTITYTYPE_NODES:
break;
case HM_ENTITYTYPE_VECTORCOLS:
break;
case HM_ENTITYTYPE_VECTORS:
break;
case HM_ENTITYTYPE_MATS:
return(HM_getMaterials);
case HM_ENTITYTYPE_PROPS:
break;
case HM_ENTITYTYPE_COMPS:
break;
case HM_ENTITYTYPE_GROUPS:
break;
case HM_ENTITYTYPE_ELEMS:
break;
case HM_ENTITYTYPE_LOADCOLS:
break;
case HM_ENTITYTYPE_EQUATIONS:
break;
case HM_ENTITYTYPE_LOADS:
break;
case HM_ENTITYTYPE_GEOMETRY:
break;
case HM_ENTITYTYPE_LINES:
break;
case HM_ENTITYTYPE_SURFS:
break;
case HM_ENTITYTYPE_POINTS:
break;
case HM_ENTITYTYPE_ASSEMS:
break;
case HM_ENTITYTYPE_CURVES:
break;
case HM_ENTITYTYPE_PLOTS:
break;
case HM_ENTITYTYPE_BLOCKS:
break;
case HM_ENTITYTYPE_TITLES:
break;
case HM_ENTITYTYPE_SETS:
break;
case HM_ENTITYTYPE_OUTPUTBLOCKS:
break;
case HM_ENTITYTYPE_LOADSTEPS:
break;
case HM_ENTITYTYPE_SENSORS:
break;
case HM_ENTITYTYPE_DESIGNVARS:
break;
case HM_ENTITYTYPE_BEAMSECTCOLS:
break;
case HM_ENTITYTYPE_BEAMSECTS:
break;
case HM_ENTITYTYPE_OPTITABLEENTRS:
break;
case HM_ENTITYTYPE_OPTIFUNCTIONS:
break;
case HM_ENTITYTYPE_OPTIRESPONSES:
break;
case HM_ENTITYTYPE_DVPRELS:
break;
case HM_ENTITYTYPE_OPTICONSTRAINTS:
break;
case HM_ENTITYTYPE_DESVARLINKS:
break;
case HM_ENTITYTYPE_OBJECTIVES:
break;
case HM_ENTITYTYPE_CONTROLVOLS:
break;
case HM_ENTITYTYPE_MULTIBODIES:
break;
case HM_ENTITYTYPE_ELLIPSOIDS:
break;
case HM_ENTITYTYPE_OPTICONTROLS:
break;
case HM_ENTITYTYPE_OPTIDSCREENS:
break;
case HM_ENTITYTYPE_TAG:
break;
case HM_ENTITYTYPE_MBJOINT:
break;
case HM_ENTITYTYPE_MBPLANE:
break;
case HM_ENTITYTYPE_DOBJREFS:
break;
case HM_ENTITYTYPE_CONTACTSURFS:
break;
case HM_ENTITYTYPE_CONNECTORS:
break;
case HM_ENTITYTYPE_SYMMETRYS:
break;
case HM_ENTITYTYPE_HANDLES:
break;
case HM_ENTITYTYPE_DOMAINS:
break;
case HM_ENTITYTYPE_SHAPES:
break;
case HM_ENTITYTYPE_SOLIDS:
break;
case HM_ENTITYTYPE_MORPHCONSTRAINTS:
break;
case HM_ENTITYTYPE_HYPERCUBES:
break;
case HM_ENTITYTYPE_DDVALS:
break;
case HM_ENTITYTYPE_BAGS:
break;
case HM_ENTITYTYPE_MAX:
break;
}
break;
case HMIN_ENTITYCLOSEFUNCTION:
break;
case HMIN_NAMEFUNCTION:
break;
case HMIN_MOVEFUNCTION:
break;
case HMIN_COLORFUNCTION:
break;
case HMIN_ASSOCIATEFUNCTION:
break;
case HMIN_CEDATAFUNCTION:
break;
case HMIN_METADATAFUNCTION:
break;
case HMIN_CLOSEFUNCTION:
break;
}
return(NULL);
}
int HM_getMaterials()
{
/* This function writes each material data structure to HyperMesh. */
int i;
//Write each material data structure to HyperMesh
for (i=0; i<nummaterials; i++)
{
HMIN_material_write(material[i].id, material[i].name);
HMIN_writeattribute_int(HM_ENTITYTYPE_MATS, material[i].id, 1, 0, 1, 1);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 2, 0, 1, material[i].E);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 3, 0, 1, material[i].G);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 4, 0, 1, material[i].NU);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 5, 0, 1, material[i].RHO);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 6, 0, 1, material[i].A);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 7, 0, 1, material[i].TREF);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 8, 0, 1, material[i].GE);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 9, 0, 1, material[i].ST);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 10, 0, 1, material[i].SC);
HMIN_writeattribute_double(HM_ENTITYTYPE_MATS, material[i].id, 11, 0, 1, material[i].SS);
}
return(0);
}