Gse
Model ElementGse is an abstract modeling element that defines a generic dynamic system. The dynamic system is characterized by a vector of inputs u, a vector of dynamic states x, and a vector of outputs y. The state vector x is defined through a set of differential equations.
Class Name
Gse
Description
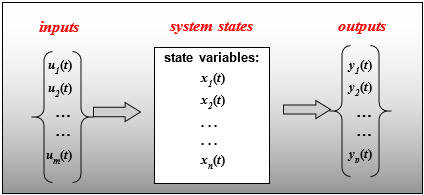
Figure 1.
Attribute Summary
Name | Property | Modifiable by command? | Designable? |
---|---|---|---|
id | Int () | No | |
label | Str () | Yes | |
no | Int () | ||
ns | Int () | ||
x | Reference ("Array") | Yes | |
y | Reference ("Array") | Yes | |
u | Reference ("Array") | Yes | |
ic | Reference ("Array") | Yes | |
static_hold | Bool () | Yes | |
implicit | Bool () | ||
function | Function ("GESSUB") | Yes | |
routine | Function () | ||
active | Bool () | Yes |
Usage
# Defined in a compiled user-written subroutine
Gse (no=int, function=userString, routine=string optional_attributes)
# Defined in a Python function
Gse (no=int, function=userString, routine=functionPointer optional_attributes)
Attributes
- no
- Integer
- function
- String
- routine
- String
- no
- Integer
- function
- String
- routine
- Pointer to a callable function in Python
- id
- Integer
- label
- String
- u
- Reference to an Array object of type U.
- y
- Reference to an Array object of type Y.
- x
- Reference to an Array object of type X,
- ic
- Specifies the Array used to store the initial values of the states, x of this GSE. You can use the ARYVAL() function with the id of this Array to access the states in a MotionSolve expression. You can also use this ID in SYSFNC and SYSARY to access the initial state values from a user subroutine.
- static_hold
- Boolean
- implicit
- Boolean
- active
- Bool
Example
- Use a GSE to define the LuGre model of
friction.
################################################################################ # Model definition # ################################################################################ def sliding_block (out_name): m = Model (output=out_name) # Model units, Gravity and Integrator settings units = Units (mass="KILOGRAM", length="METER", time="SECOND", force="NEWTON") grav = Accgrav (jgrav=-9.800) gstiff = Integrator (error=1e-5) # Points and Vectors that will be reused p0 = Point (10,0,0) ux = Vector (1,0,0) uy = Vector (0,1,0) uz = Vector (0,0,1) # Ground part and global coordinate system grnd = Part (ground=True) oxyz = Marker (body=grnd, label="Global CS") # Block blk = Part (mass=1, ip=[4.9e-4,4.9e-4,4.9e-4], label="Block") blk.cm = Marker (body=blk, qp=p0, zp=p0+uz, xp=p0+ux, label="Block CM") # Translational joint between Block and Ground along global X-axis im = Marker (body=blk, qp=p0, zp=p0+ux, xp=p0+uz, label="Joint Marker on Ground") jm = Marker (body=grnd, qp=p0, zp=p0+ux, xp=p0+uz, label="Joint Marker on Ground") jnt = Joint (type="TRANSLATIONAL", i = im, j = jm, label="Trans Joint") # An external force trying to move the block sfojm = Marker (body=grnd, qp=p0, zp=p0+ux, xp=p0+uz, label="Sforce reaction") sf = Sforce (type="TRANSLATION", actiononly=True, i=im, j=sfojm, label="Actuation Force", function = "3*sin(2*pi*time)") # Friction in the joint m.lugre = LuGre(joint=jnt) # Some requests of interest m.r1 = Request (type="DISPLACEMENT", i=im, j=jm, rm=jm, comment="Joint displacement") m.r2 = Request (type="FORCE", i=im, j=jm, rm=jm, comment="Joint forces") m.r3 = Request (type="VELOCITY", i=im, j=jm, rm=jm, comment="Joint velocity") so = Output (reqsave=True) # Return the model you just defined return m ############################################################################### ############################################################################### ############################################################################### m = sliding_block("lugre1") m.simulate (type="DYNAMICS", end=4, dtout=.01) # Change the static friction coefficient and continue simulation m.lugre.mus=0.5 m.simulate (type="DYNAMICS", end=8, dtout=.01)
Comments
- See Properties for an explanation about what properties are, why they are used, and how you can extend these.
- For a more detailed explanation about Gse, see Control: StateEqn