HM_ExtAPI::CreateGeomFaceDirect()
Creates face in HyperMesh database.
Syntax
bool CreateGeomFaceDirect(
HM_EntityGeomFace& new_face,
const HM_EntityGeometrySurface& base_surf,
int number_of_loops,
const int* loop_coedge_counts,
const HM_EntityGeometryCurve* loop_coedge_curves,
const double* curve_start_params,
const double* curve_end_params,
const bool* coedge_curve_dirs,
bool surf_sense,
HM_EntityGeomCoedge** result_coedges,
HM_EntityComponent comp
);
Type
HyperMesh Ext API Function
Description
Created face must have at least one loop that defines its external boundary in parametric space.
If the function succeeds, the return value is true. If the function fails, the return value is false. To get extended value information, call HM_ExtAPI::GetLastErrorCode().
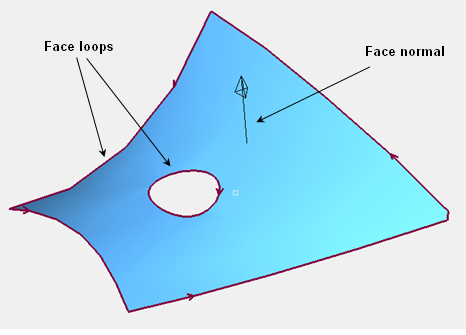
Figure 1.
In parametric space, the direction of loops depends on geometric normal use defined by the parameter surf_sense. In case if the value of the parameter surf_sense is true then the face normal is oriented in the same direction as geometric surface normal and the "face on the left" rule applies in parametric space as well. The external loop of the face is oriented counter clockwise while all internal loops are oriented clockwise.
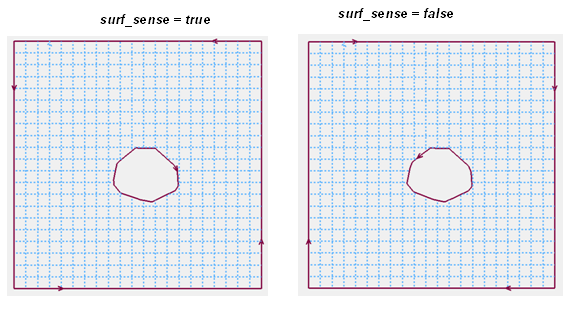
Direction of the geometric normal of the surface is defined by the surface parameterization function S(U, V) as the direction of the cross-product of parametric derivatives:
N = ∂S(U, V)/∂U X ∂S(U, V)/∂V
The parameter loop_coedge_curves points to the array of curve handles corresponding to curves used to construct loop coedges. Each curve is interpreted as parametric curve. That is, the x and y coordinates of each curve point is used as, respectively, U and V coordinates in parametric surface space. The z coordinate of the curve points is ignored.
The array contains curves for all loops. The order of the curves for each loop is defined by the direction of the loop. The curves for the coedges of the external loop must appear first in the array.
Both curve_start_params and curve_end_params must be NULL or not NULL at the same time. If both parameters are NULL, then default parametric range of each curve is used to create the coedge. Use the function HM_ExtAPI::GeomCurveGetParameterBounds to obtain curve parametric range.
To free arrays allocated for result_coedges during the function call use the function HM_ExtAPI::MemoryFree().
Requires including hm_extapi.h.
Inputs
- new_face
- [out] - Handle to new face object.
- base_surf
- [in] - Handle to surface objects that was returned by previous calls to API functions.
- number_of_loops
- [in] - Number of face loops.
- loop_coedge_counts
- [in] - Pointer to the array that contains number of coedges in each loop. The number of entries in the array must equal number_of_loops.
- loop_coedge_curves
- [in] - Pointer to array of curve handles returned by previous calls to API functions. Each handle represents the curve in 2D parametric space of the surface base_surf and corresponds to one of coedges defining face loops. The number of entries in the array must equal to the sum of loop coedge counts given by the array loop_coedge_counts.
- curve_start_params
- [in] - If not NULL, then points to the array of values that define lower parametric bound of corresponding curve from the array loop_coedge_curves used to create the coedge. The number of entries in the array must equal to the number of entries in the array loop_coedge_curves.
- curve_end_params
- [in] - If not NULL, then points to the array of values that define upper parametric bound of corresponding curve from the array loop_coedge_curves used to create the coedge. The number of entries in the array must equal to the number of entries in the array loop_coedge_curves.
- coedge_curve_dirs
- [in] - If not NULL, then points to the array of flags that define the direction of the curves given by loop_coedge_curves with respect to the loop direction. The value of true indicates that the direction of the curve within the coedge is the same as the loop direction. The value of false indicates that the direction of the curve is opposite to the direction of the loop. If the parameter is NULL, then the value of true is assumed for all curves.
- surf_sense
- [in] - Defines normal direction of created face. The parameter can have one of following values.
- result_coedges
- [out] - If not NULL, then returns pointer to the array of coedges created for each of the curve in the input array. The number of entries in the array is the same as in the input array loop_coedge_curves.
- comp
- [in] - If not NULL, then component where the face is created. If NULL, then the line is created in current component.
Example
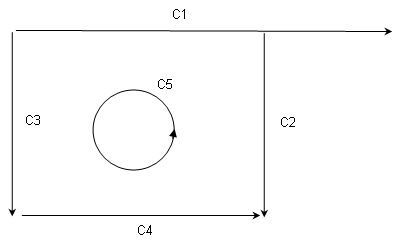
The curves C1, C2, C3, C4 are straight lines, for which the segments corresponding to parametric range 0 … 1 are shown. The curve C5 is a circle with angular parametric range 0… 2π.
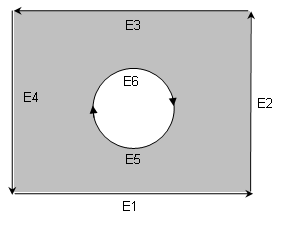
That is, your intent is to create a face with the normal oriented in the same direction as the normal of geometric surface, and we want to create two loops. Note also that your intent is to split the internal circular loop into two edges.
You will need to provide arrays with curve parameters (curve_start_params and curve_and_params) because we need to cut the curves C1 and C5. You also will need to provide an array of curve direction flags because the curves C5, C1 and C2 have direction opposite to the direction of the loops that you need to have as a final result.
number_of_loops | 2 |
loop_coedge_counts | 4, 2 |
loop_coedge_curves | C4, C2, C1, C3, C5, C5 |
curve_start_params | 0, 0, 0, 0, π, 0 |
curve_end_params | 1, 1, 0.7, 1, 2π, π |
coedge_curve_dirs | true, false, false, true, false, false |
surf_sense | true |
Errors
None.